Node.JS is JavaScript on a server. It hangs on a single thread, using non-blocking I/O queries. Thanks to this, it can process tens of thousands of connections at the same time. It has advantages, but it also has flaws. It’s certainly not for everyone. D’uh!
But if you have a minute to read more about it… You might ask if JavaScript is also for the backend. But let’s start from the beginning.
Node.js is a lightweight solution for real-time apps working simultaneously on distributed devices. It’s efficient thanks to its non-blocking, event-driven I/O. It’s not exactly universal, and it won’t solve all your problems, but it’s great for fulfilling specific needs. Here’s what you have to need.
Node.js was laughing stock back in the day
Know thy enemy, they say, and know thy friend even better. Node.js was created in the ancient time of 2009 when JavaScript was used mostly for internet funnies, and the idea of having it on a server was considered a bad joke.
However, once upon a time Ryan Dahl got really annoyed. It was when he couldn’t see the progress of uploading his photos to Flickr. This was the spark that inspired him to create Node.js, a simple solution that anyone can implement. It quickly gathered a following and was used in the following projects.
- 2010 – Express and socket.io use Node.js based solutions
- 2011 – Hapi, LinkedIn, and Uber express their interest in Node.js
- 2013 – Koa and Ghost (the first JS-based blog platform) are created
- 2015 – Node.js Foundation is established
- 2016 – Yarn, Node.js 6
- 2017 – Node.js 8, HTTP/2, 3 BILLION downloads weekly
- 2018 – Node.js 10
- 2019 – Node 12
- 2020 – Node 14 & 15
Now, why should YOU have any interest in Node.js?
To answer this, let’s establish the foundation. A typical application consists of:
- Front-End
- Back-End
- Database
Now, each of these elements requires professionals proficient in different languages. That’s costly. And unnecessary, considering that you can take care of all of them with just a JavaScript team. If they know their way around Node.js, you can connect with a MySQL base, or include such stuff in the backend as Python, Ruby, C Sharp. For the database, you can use Postgresql, Oracle, or NoSQL. Easy!
Here’s what JavaScript offers you today – and what hadn’t been possible until Node.js
- Access to a server-based file system
- Operations on files
- Advanced support for web protocols
- JavaScript running as a service on a server
- Sharing the code and package managers
That’s quite an achievement, and it was made possible with Node.js, a JavaScript runtime built on Chrome’s V8 JavaScript engine. Its package ecosystem, npm, is the world’s largest ecosystem of open source libraries.
The main problem of JavaScript, at first, was that nothing was truly compatible with it. Browsers were—in theory. But in practice, well, you know…
However, Chrome’s engine has this little thing called V8, with an open-source code. It’s lightning-fast and it’s meant to work with JavaScript! The same engine is used in Node.js. Written in C++, it allows you to compile JavaScript to a machine language, which is interpreted in real-time.
Another thing that makes Node.js so effective and fast is the event-driven, non-blocking I/O model.

You could say that Node.js combines all the goodness that JavaScript delivers when it comes to I/O operations. Here’s how it works in its asynchronous glory.
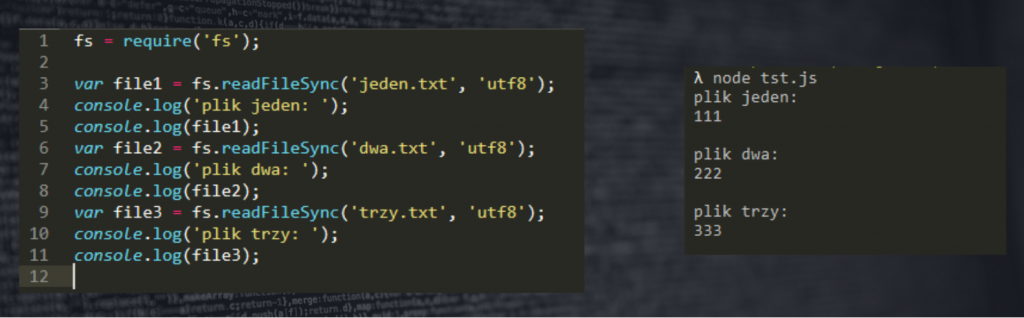
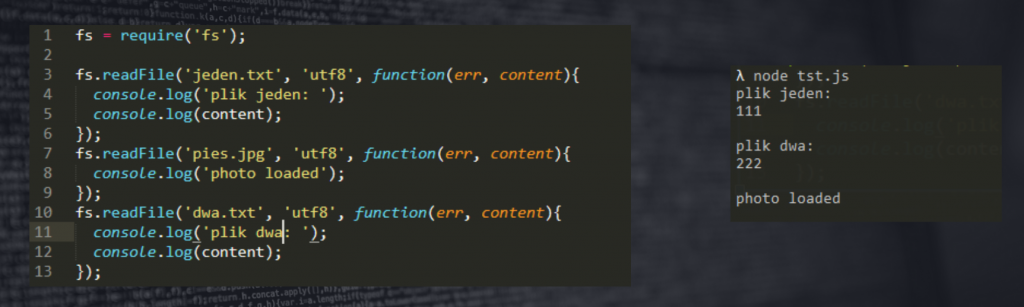
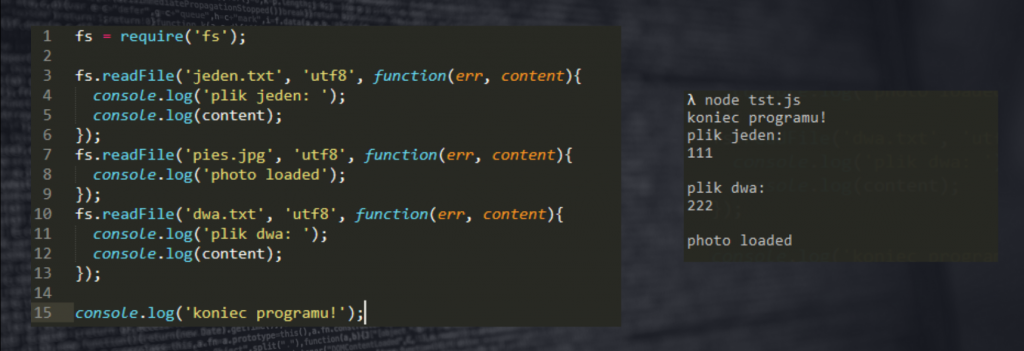
NPM
The package ecosystem you can dive into via the Node Package Manager (NPM) includes millions of frameworks and libraries as well as utils, bootstrap, jquery or react. More than 11 million JavaScript developers use the NPM to make managing libraries easier in their development project.
Let’s take a look at Node.js actually working. Here’s a shortlist of frontend solutions with Node in the background:
- Frameworks that support developing backed apps in JavaScript (Express, Sails)
- Bundlers that combine dependencies into a single whole (Webpack)
- Test-runners that enable you to test the code (Mocha, JEST, Jasmine, Karma, Puppeteer)
- Linters and tools for static code analysis (ESLint)
- Tools that support the projects from the console level (Angular CLI, Svelte CLI)
Now let’s see who uses Node.js!
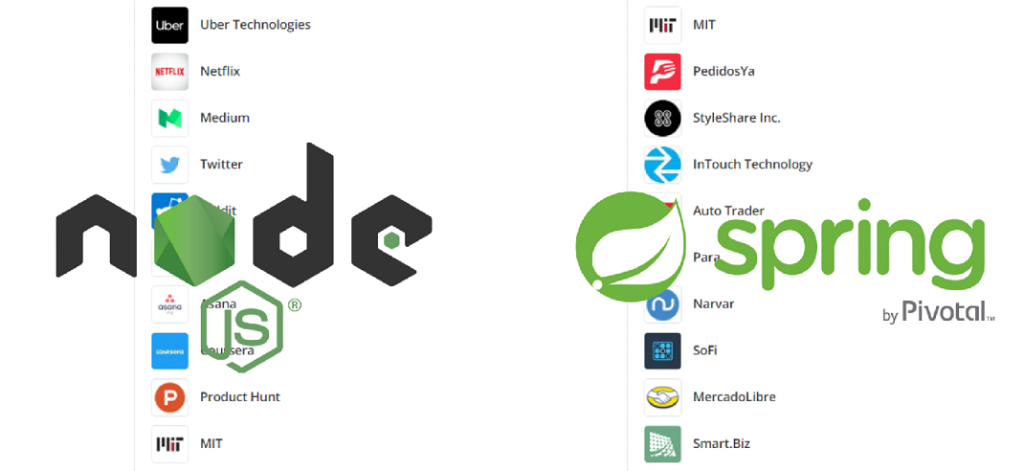
Everyone knows the brands from the left column, right? That’s because the giants really like Node.js. It’s because it allowed them to achieve some outstanding feats.
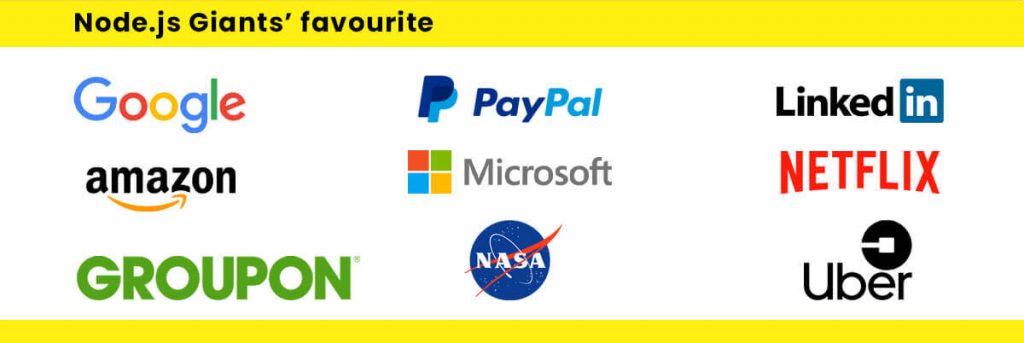
You already know why technical folks fall in love with Node.js. Now it’s time to explain, how Node.js has been working for these big companies:
- Reducing the number of hosting devices 10x
- Reducing the number of servers from 30 to 3
- Doubling the traffic capacity
- Increasing the performance significantly and lowering the memory use
- Increasing the speed of the new mobile app up to 20x in some scenarios
- Merging the frontend and backend teams
- Getting a safety margin that allows supporting 10x the resource usage
Uber
- Quick and flawless processing of extremely large amounts of data
- Eliminating the errors without having to restart
- Quick implementation of new code
- Accessing the strong open source community to develop new solutions with
- Quickly responding to changing business needs
PayPal
- Building a Node.js app two times faster and with a smaller team than on the previous Java-based app
- Doubling the query-per-second rate
- Reducing the average server response time by 35%
- Reducing the code length by 33%
- Reducing the number of files by 40%
Netflix
- Reducing the app opening time by 70%
- Improving further development and shortening the time to create a new version of the app
- Reducing the environment launch time from 40 minutes to under 1 minute
NASA
- Reducing the number of steps in the process from 28 to 7
- Moving all the data from the cloud to the corporate architecture of Node.js
- Using a single database for everything
- Reducing the time needed to access the data by 300%?!?!?!?!?!?!?!
- Switching to Node.js for designing the EVA suit
Trans.info
(it’s the largest information portal about the transport industry in Europe, we wrote more about our contribution to the project here)
- Improved visibility in Google due to SSR
- Reducing the page load time from 12 to 3 seconds
- Increasing the control over the application
- Server-based authentication
- Uninterrupted operation of the application during deployment, making it invisible to the user
And here’s the simplest HTTP server in Node.js
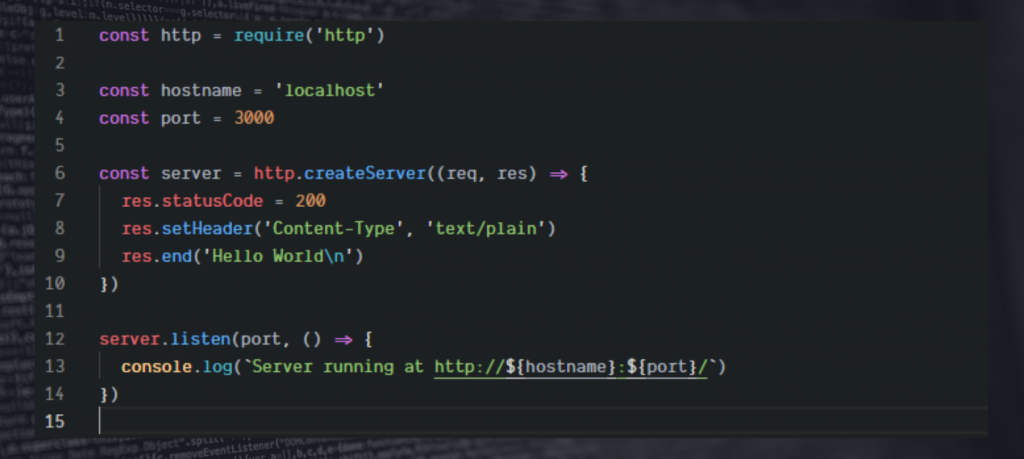
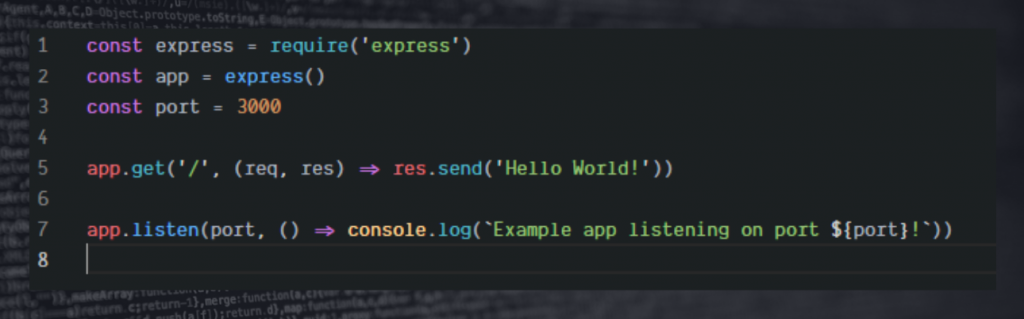
Node.js support for template engines includes the following:
- EJS (the most frequently used one)
- Handlebars
- PUG
- Haml.js
- Blade
- React
So why would you want to use Node.js?
- It’s scalable and well-performing
- It’s versatile
- It offers fast development
- You can use a proxy server
- You can effectively use your code
- It eliminates the need to use other programming languages
- It has a non-blocking I/O process
- You can implement changes without interruptions
- Processing is incredibly smooth
- Data streaming is way more effective, as proven by Netflix
- Testing new functionalities becomes quick and easy
- You can have one team taking care of frontend and backend
- It’s open-source, with countless libraries in the NPM
What will be the challenges?
- You have to work out the ORM transactions
- It requires technical prowess. The more experienced your programmers, the faster your cases get done
- This is an open-source project and there are many solutions and you must choose one correct
- There is no framework like Spring
- The client environment must be well prepared for metrics
Node.js for enterprise applications?
The Node.js runtime environment is becoming a popular choice for developing server-based apps. But is it good for enterprise projects, which tend to be more complex? It is used for microservices, after all.
Most organizations need two groups of IT people. One to take care of traditional IT operations, encompassing CRM, ERP, mainframe apps, etc. And one to research and develop next-gen apps that require dynamic changes. For them, Node.js is a viable option. But only for them. For the first group, you really need something easy-going and predictable.